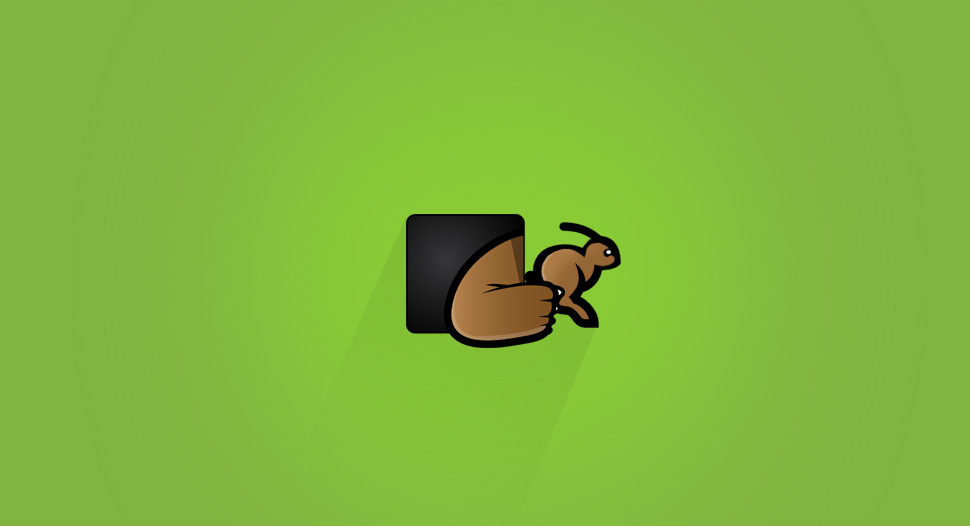
Escaping theme rejection
Growth in numbers of malicious or hacked websites often reminds us, how important security is.
As a theme or plugin developer, your clients and users expect you to know how to write secure code. The rule of a thumb of secure coding is always sanitizing input and always escaping output. If you plan to publish your theme, either on ThemeForest or on wordpress.org, you will need to follow this rule strictly, or your theme/plugin will be rejected.
“A web is a dangerous place.”
Said a fly before eaten by a spider.
While hurrying to get the job done, developers often don’t pay enough attention to this. Honestly, our themes also got rejected in the past on Themeforest due to data validation.
WordPress has some handy helper functions, that help with escaping. You should use them every time before echoing. Let’s see how it’s done.
Escape HTML
We should use esc_html() function to escape every data we are outputing.
<div class="anps-desc"><?php echo esc_html($description); ?></div>
Escape textarea
esc_textarea() is using for text inside textarea tags. It is almost identical as esc_html(), difference is that esc_textarea() double encode entities.
<textarea><?php echo esc_textarea($content); ?></textarea>
Escape attributes
esc_attr() is the same as esc_html() function, but you should to use for all HTML element’s attributes.
<tr class="<?php echo esc_attr($class); ?>">
Escape urls
You also need to escape every url, which is added dynamically (with PHP). It is also important not to escape only href inside a element, but also src inside image element.
<img src="<?php echo esc_url($image_src); ?>" alt="<?php echo esc_attr($image_alt); ?>">
Escape javascript
If you are using inline javascript inside html, than you also need to make it safe by adding esc_js() function.
<a href="#" onclick="<?php echo esc_js($my_js); ?>"></a>
Escape translations
All translation strings also have to be escaped. We used in our themes __() and _e() functions, but these functions are not safe enough, so you should esc_html__() and esc_html_e() instead. There are also option to use esc_attr__() and esc_atr_e(), especially if you have translations inside html attributes.
<?php echo '<li>' . esc_html__('Search results', 'construction') . '</li>'; ?>
Escape multiple code lines at once
In some cases you won’t be able to use any of examples above, escpecially if you store html data to one php variable and than just “echo” it later.
$output = "<div class='color-input'>";
$output .= "<label for='$option_name'>$label</label>";
$output .= "<input data-value='$color_option' class='color-pick-color' readonly>";
$output .= "<input class='color-pick' type='text' name='$option_name' value='$color_option' id='$option_name'>";
$output .= "</div>";
In this example we use wp_kses() function.
echo wp_kses($output, array(
'div' => array(
'class'=>array()
),
'label' => array(
'for' => array()
),
'input' => array(
'data-value' => array(),
'style' => array(),
'class' => array(),
'readonly' => array(),
'type' => array(),
'name' => array(),
'value' => array(),
'id' => array()
)
));
Conclusion
To escape soft reject and also make your code safe (avoid XSS and malformed HTML), it is very important to use those WordPress helper functions.